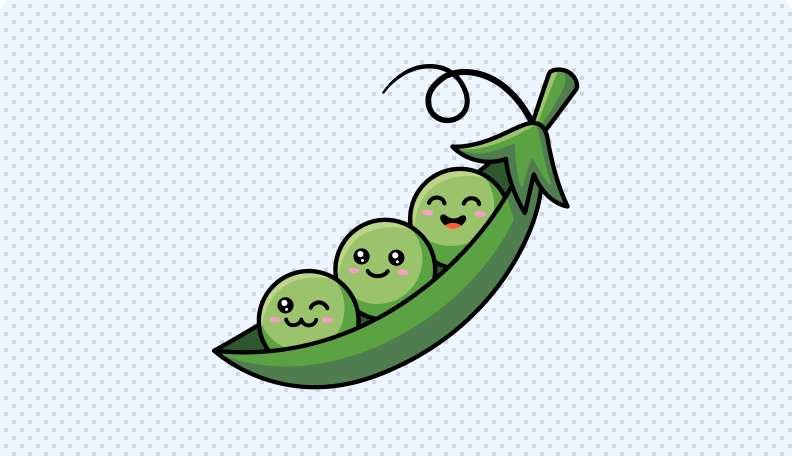
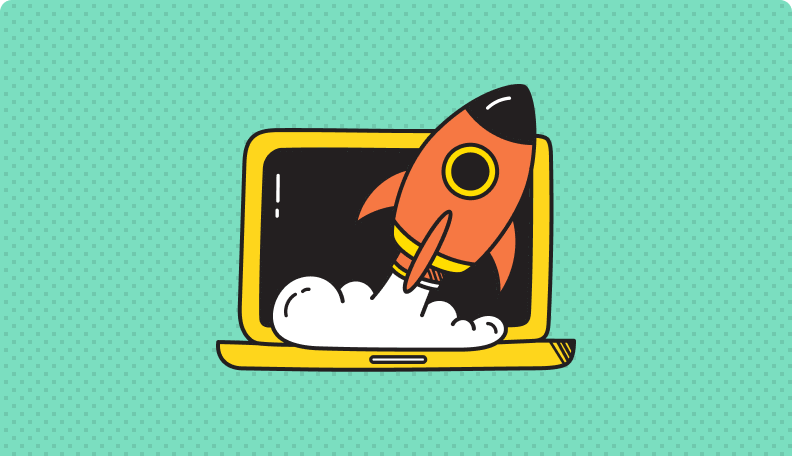

At first glance, you might assume that an operator is a way to implement mathematical or logical functions in Kubernetes – because that's what operators do in the context of programming languages. But actually, Kubernetes operators have nothing to do with programming or math. Instead, they're used mainly to package and deploy applications.
We don't know why the Kubernetes developers chose to name them operators. But we do know all about how they work, when to use them, and best practices for working with them – all of which we cover in this blog post.
What is a Kubernetes operator?
A Kubernetes operator is a way to manage applications or other resources in Kubernetes using custom resource definitions and the Kubernetes API.
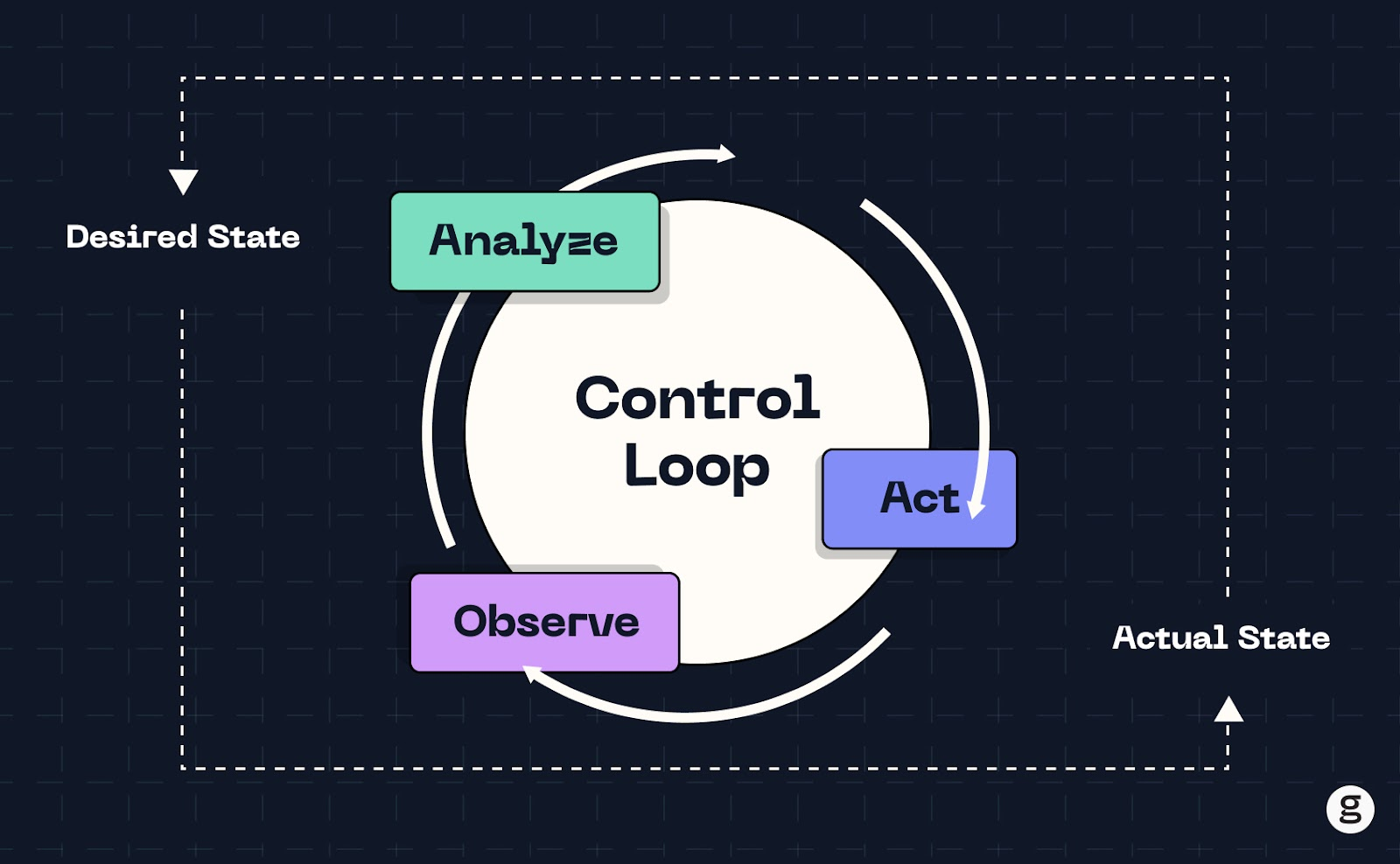
Operators are a type of controller, a native component of Kubernetes that the control plane uses to manage various types of objects and resources (like Deployments). All Kubernetes controllers work by monitoring the actual state of a resource, comparing it to a desired state defined by an admin, and trying to make the actual state match the desired state. Operators do this by comparing a desired application deployment to the actual state of the cluster, and then bringing the two states into alignment – which effectively deploys an application.
That said, what makes operators unique from other Kubernetes controllers is that operators don't monitor any objects that are built into Kubernetes by default. Instead, they focus on custom resources. By implementing custom resources that need to be present to deploy an application, operators enable application deployment.
Kubernetes Operator vs. Helm vs. Controller
We just went over the basics of how operators relate to controllers. But let's dive a little deeper by comparing operators to Helm charts and controllers. Each of these methods can be used to deploy applications in Kubernetes, but they work in different ways and are suited to different use cases.
Operators vs. Helm charts
Helm charts are a way of installing Kubernetes applications using Helm, a package manager. Compared to operators, Helm charts are less flexible and customizable, but they are also simpler.
If a Helm chart for an application you want to deploy already exists, and you don't need to customize the app, using Helm to install it is probably fine. But for more complex application deployment scenarios – or for situations where you're not deploying a new app, but instead performing a task like application backup, which Helm doesn't support – operators are preferable.
For more details, check out our Kubernetes operator vs Helm blog.
Operators vs. controllers
As we mentioned above, operators are a type of controller, so they're not actually distinct things. However, operators are only one of several controllers available in Kubernetes, and unlike the other "native" controllers, operators don't manage resources that are built into Kubernetes by default. That's why operators require custom resource definitions, or CRDs.
You can deploy an application using other types of controllers – like the Deployment controller, which lets you run applications by creating a manifest that tells Kubernetes which Pods and containers to run. If your application is relatively simple and only requires some basic configuration parameters, a Deployment is usually the simpler way to get it up and running. But complex apps – like those with complicated dependency lists or internal networking requirements – may require operators because they depend on parameters that are difficult or impossible to configure using a Deployment.
Kubernetes Operator examples
If you're looking for examples of Kubernetes operators, OperatorHub.io is your go-to resource. It hosts a large repository of operators that are freely available to download and install.
There are far too many operator examples to cover here, but to provide a sense of which types of apps or tools you might install with an operator, consider these samples:
- Istio, the open source service mesh.
- The Container Security Operator based on Project Quay, an open source container image scanner.
- Apache Spark, the open source clustered computing platform.
- An operator that integrates Kubernetes with Amazon CloudWatch for logging purposes.
It's worth noting that in many cases, apps that you can install with operators can also be installed using Helm charts. (For example, Istio supports Helm as an installation method.) You could also install some of these apps as basic Deployments if you wanted. This drives home the point that operators aren't totally unique; there are overlapping functionality and use cases between operators, other controllers, and Helm.
But in general, examples of applications that require or encourage installation via operators are more complex apps that would be challenging to deploy using other methods.
Benefits of Kubernetes Operators
We've hinted already at which benefits operators offer over other types of Kubernetes application deployment methods, but let's discuss those advantages in more detail.
The key benefits of Kubernetes operators include:
- Automation: Operators can help to automate complex tasks (like configuring complicated networking setups) that would otherwise be tedious to implement.
- Flexibility: Because operators operate based on custom resource definitions, they provide an open-ended way to configure resources. You're not constrained by the capabilities of built-in controllers, as you would be when using Deployments, for example.
- Shareability and reusability: In most cases, the code that powers an operator can be easily shared between Kubernetes clusters. This makes operators a convenient way of distributing applications for multiple users.
In short, operators offer the benefit of a flexible, extensible approach to deploying applications, as well as performing other tasks that involve complex changes to resource configurations in Kubernetes.
How to create a Kubernetes operator
To create a new Kubernetes operator from scratch, you need to complete two main tasks:
- Defining custom resources for the operator to interact with. You'd typically use YAML for this purpose.
- Writing operator code that tells the operator how to make requests to the Kubernetes API. You can do this using any programming language supported by the Kubernetes API.
Note, however, that most people these days don't develop operators completely from scratch. Instead, they rely on toolkits or SDKs that help to automate the process, such as the Operator Framework.
Kubernetes Operator Framework
As an example of how to write an operator using an SDK or framework, here's a quick look at how the Operator Framework helps you.
After installing the Operator Framework, you can define how your operator interacts with the Kubernetes API using a simple operator-sdk create api command. This saves you from having to write a bunch of code from scratch. You'll still need a custom resource definition, but that's typically much easier to generate.
For details of what it takes to develop an operator using the Operator Framework, check out tutorials like this one on creating Go-based operators.
Best practices for writing Kubernetes operators
If you choose to create your own operator, the following guidelines can help make it as usable and secure as possible.
Be sure you really need an operator
The first best practice for writing operators is ensuring that you don't write an operator when a better method – like Helm – would meet your needs. Operators are really only necessary when you're dealing with truly complex application deployments. We haven't surveyed Kubernetes admins about their preferences, but we'd bet that if you did, you'd find that most prefer using Helm over operators because Helm is simpler to work with in many respects.
So, if you can meet your requirements without using operators, you'll probably save a lot of time – and make life easier for anyone who will be installing your application – if you stick with a simpler deployment technique.
Create one operator per requirement
The open-ended nature of operators means that it's technically possible to have one operator try to do multiple things – like install two apps at once. This is not a best practice in most cases, however. Trying to cram too much functionality into one operator increases your risk of making mistakes. It also makes it harder to manage applications because you may want to install just one app, which you can't do if your operator installs other apps as well.
It's better to have each operator do one thing, and do it well.
Keep CRDs simple
When creating custom resource definitions for an operator, stick to the basics and avoid unnecessary parameters. This not only makes operators easier to write, but also reduces your risk of introducing changes that accidentally break something.
Test your operators
Because operators can manage custom resources via the Kubernetes API, mistakes within operators could lead to big performance or stability issues for your Kubernetes cluster. For that reason, it's a best practice to test operators carefully within a dev/test Kubernetes cluster before deploying them into production.
Validate the origins of third-party operators
The fundamentals of software supply chain security apply to operators just as they do to any type of application packaging solution. If you download a third-party operator from a public repository, make sure you trust the developers who provided it.
Make sure, too, that you obtained the operator from an official, trusted source – as opposed to a malicious repository where someone uses typosquatting to try to trick people into downloading tainted operators.
Consider an operator SDK
As we mentioned, creating an operator totally from scratch is a lot of work. In general, using an operator SDK will not only save you time, but also help you generate operators that are more consistent in how they work (because SDKs enforce conventions in operator design and implementation that you may not follow if you were to write your own operator from the ground up).
The only reason not to use an operator SDK is if you’re creating a very unusual application that requires custom Kubernetes API functionality or other capabilities that are not supported by any operator frameworks or SDKs – but that's rare today, given the maturity of operator toolkits.
How to install and remove Kubernetes operators
Once you have an operator – either because you created it yourself or you obtained it from a repository like OperatorHub – you can install it by simply applying its custom resource definition using a command like:
To remove an operator, you can delete its custom resource definition using a command such as:
Managing operators with Operator Lifecycle Manager
If you work with operators frequently, you may wish to install a tool like Operator Lifecycle Manager, or OLM. OLM automates much of the work required to find, install, and remove operators, providing an experience that is akin to using a standard package manager.
Troubleshooting operators
If something goes wrong when deploying or managing an operator, your first move should be to check the status of the resource that you deployed using the operator. You can do this using kubectl:
If the resource is not running, it's likely that the operator didn't deploy properly. In that case, you can check any log files that exist for your operator. Whether logs are available depends on whether the operator developer implemented logging. Log file location also depends on how logs were implemented.
If you can't access logs, you can try to troubleshoot further by attempting to deploy the operator on a different Kubernetes cluster. This will help indicate whether the issue is related to your cluster configuration, or is a problem with the operator itself.
You may also have more success by redeploying the operator using a framework like OLM, which we mentioned above. That method may generate more feedback about why the operator is not working.
If all else fails, check your observability platform for Kubernetes alerting and Kubernetes monitoring insights. These may alert you to problematic cluster behavior like exhaustion of cluster resources, which could cause operators to fail even if there isn’t a problem with the operator itself.
How to Use Kubernetes Operators with groundcover
This brings us to how operators and groundcover fit together. By providing comprehensive Kubernetes observability insights, groundcover helps ensure that you know when something's going awry with your clusters that may interfere with operator behavior – to mention just one of the many benefits that groundcover delivers.
You can also use operators alongside groundcover to enable operator-based metrics, a method that uses CRDs to scrape targets.
Operators all the way
To be sure, operators aren't always the best way to manage resources in Kubernetes. We love Helm as much as everyone else, and we're not here to tell you to opt for operators over Helm charts. But when you have a complex application or use case, operators are typically the way to go. They provide unparalleled levels of control that allow you to extend Kubernetes's functionality in powerful ways.